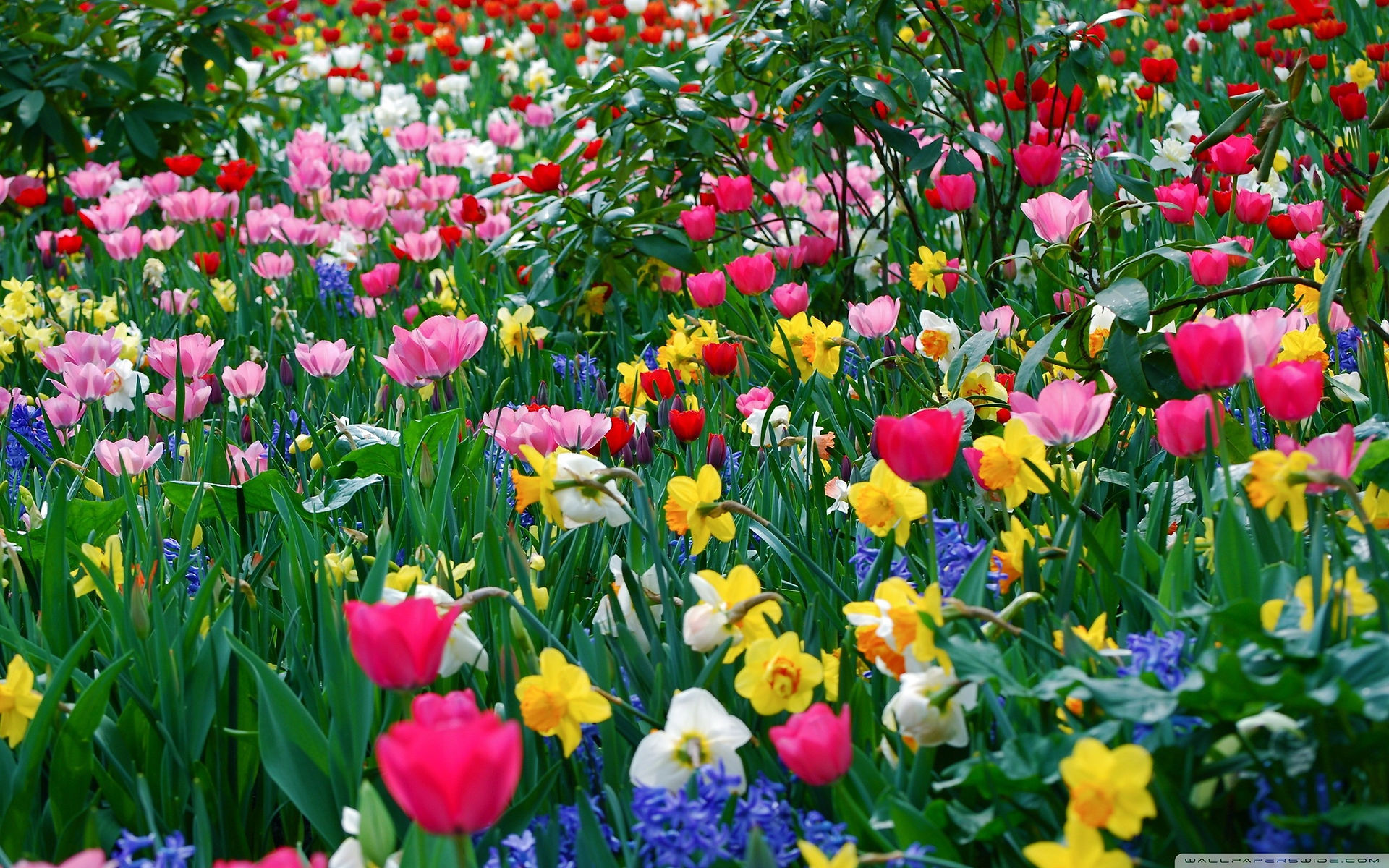
Coding with Python
Vocabulary
​
Program or Code - a set of instructions telling the computer what to do
Function: Tells the computer what to do, Example: print
Algorithm: set of steps, in order, to complete a task
Pseudocode or flowcharts: Used to plan for writing code
-
Pseudocode: Just written steps to plan
-
Flowcharts: a visual diagram for steps using different shaped boxes
Chapter 1
Data: Stored information
Variables: where data is stored
-
Variable rules:
-
No spaces or special characters
-
Cannot start with a number
-
Cannot use a Python function, example: print
-
Value: content of box where data is stored
String: stored text
Integers: whole numbers
Floats: decimal numbers
Comments: note written in natural language, use # symbol followed by comment (use bold text for comments)
Hello World
>>> print('hello, world')
hello, world
>>> player_score = 0 # Or use >>> PlayerScore = 0
>>> player_score # result >>> PlayerScore
0 0
>>> print(player_score)
0
>>> player_score = player_score + 1
>>> player_score
1
>>> name = 'Mustangs'
>>> message = ' like to code'
>>> name + message
'Mustangs like to code'
>>> name = input('What is your name?')
What is your name?Colleen
>>> name
'Colleen'
>>> player_score = 100
>>> username = 'Julia'
>>> print('Your final score is', player_score)
Your final score is 100
>>> print('Nice to meet you', username, '.')
Nice to meet you Julia .
Math
>>> print(23) #integers
23
>>> print(3.14) #floats
3.14
>>> print(23,end=',')
23,
>>> 350+42 #Addition
392
>>> 987-120 #Subtraction
867
>>> 34*45 #Multiplication
1530
>>> 57/2 #Division
28.5
>>> 57//2 #Floor division - discards fractional part
28
>>> 57%2 #Returns the remainder only from division
1
>>> 3**2 #3 raised to the power of 2
9
>>> round(100/3, 2) #round the result to 2 places
33.33
>>> (100 -5*3) / 5 #Order of operations
17.0
>>> width = 100
>>> height = 20
>>> area = width * height
>>> print(area)
2000
>>> distance = 102.52
>>> speed = 20
>>> time = distance / speed
>>> time
5.1259999999999994
>>> number = input("Enter a number: ") #Number treated as word
Enter a number: 2
>>> number + number #Word + Word
'22'
>>> number = int(number)
>>> number = input('Enter a number: ')
Enter a number: 2
>>> number + number
'22'
>>> number = int(number)
>>> number + number
4
Chatbot
Chatbot: a program that appears to talk intelligently to a human using text
Click on File > New File in Python 3.8.2 Shell
File > Save As
Run the code by clicking on Run > Run Module
print('Hello. I am Zyxo 64. I am a chatbot.')
print('I like animals and I love to talk about food.')
name = input('What is your name?: ')
print('Hello',name,', nice to meet you!')
# get year information
year = input('I am not very good at dates. What is the year?:')
print('Yes, I think that is correct. Thanks! ')
# ask user to guess age
myage = input('Can you guess my age? - enter a number: ')
print('Yes you are right. I am',myage,'.')
# do math to calculate when chatbot will be 100
myage = int(myage)
nyears = 100 - myage
print('I will be 100 in', nyears, 'years.')
print('That will be the year', int(year) + nyears, '.')
# food conversation
print('I love chocolate and I also like trying out new kinds of food')
food = input('How about you? What is your favorite food?:')
print('I like' ,food,'too.')
question = 'How often do you eat '+food+ '?:'
howoften = input(question)
print('Interesting. I wonder if that is good for your health?')
# animal conversation
animal = input('My favorite animal is a giraffe. What is yours?: ')
print(animal,'! I do not like them.')
print('I wonder if a', animal, 'likes to eat', food, '?')
# conversation about feelings
feeling = input('How are you feeling today?: ')
print('Why are you feeling', feeling, 'now?')
reason = input('Please tell me: ')
print('I understand. Thanks for sharing.')
# Goodbye
print('It has been a long day!')
print('I am too tired to talk. We can chat again later.')
print('Goodbye', name,'I liked chatting with you!')
Run Module
= RESTART: C:/Users/Colleen/AppData/Local/Programs/Python/Python38-32/Chatbot.py
Hello. I am Zyxo 64. I am a chatbot.
I like animals and I love to talk about food.
What is your name?: Colleen
Hello Colleen , nice to meet you!
I am not very good at dates. What is the year?:2020
Yes, I think that is correct. Thanks!
Can you guess my age? - enter a number: 13
Yes you are right. I am 13 .
I will be 100 in 87 years.
That will be the year 2107 .
I love chocolate and I also like trying out new kinds of food
How about you? What is your favorite food?:pizza
I like pizza too.
How often do you eat pizza?:weekly
Interesting. I wonder if that is good for your health?
My favorite animal is a giraffe. What is yours?: tiger
tiger ! I do not like them.
I wonder if a tiger likes to eat pizza ?
How are you feeling today?: great
Why are you feeling great now?
Please tell me: it is Saturday
I understand. Thanks for sharing.
It has been a long day!
I am too tired to talk. We can chat again later.
Goodbye Colleen I liked chatting with you!
>>>
​
Chatbot Experiment
-
Mad Libs
-
Song Lyrics Generator
-
Unit Converter
-
Restaurant Bill Calculator
-
Paint Calculator
​
Chapter 2
​
Art Masterpieces - Turtle Graphics
​
#Remember to save and Run
>>> import turtle
>>> turtle = turtle.Turtle()
>>> turtle.shape('turtle') # looks like a turtle
>>> print(turtle.xcor(),turtle.ycor())
0.0 0.0
​
>>> turtle.forward(100)
>>> turtle.right(90)
>>> turtle.left(60)
>>> turtle.backward(100)
>>> turtle.color('red')
>>> turtle.circle(10)
>>> turtle.penup()
>>> turtle.pendown()
>>> turtle.reset()
>>> turtle.goto(35,80)
>>> turtle.hideturtle()
>>> turtle.showturtle()
Loops
>>> turtle.reset()
>>> for i in range(4): # turtle will draw a square
turtle.forward(100) # variable is i but could use any name
turtle.left(90) # for i in range(1,5) also possible
print(i) # Add this NEW LINE # for i in range(1,10,2)
0
1
2
3
>>>
Adding Color
import turtle
turtle = turtle.Turtle()
turtle.begin_fill()
turtle.color('red')
for i in range(4):
turtle.forward(100)
turtle.left(90)
turtle.end_fill()
Nested Loops
turtle.shape('turtle')
# outer loop repeats the square 6 times
for n in range (6):
# inner loop repeats 4 times to make a square
for i in range(4):
turtle.forward(100)
turtle.left(90)
turtle.right(60) # add a turn before the next square
​
Data in Lists
>>> color = ["red","green","blue"]
>>> color[0]
'red'
>>> color[1]
'green'
>>> turtle.color(colors[i])
>>> for i in range(3):
turtle.color(colors[i])
turtle.forward(50)
print(colors[i])
red
green
blue
>>>
Geometric Art
import turtle
turtle = turtle.Turtle()
for i in range(6): # make a geometric pattern
turtle.forward(100) #repeat 6 times - move forward and turn
turtle.left(60)
Geometric Art using a Nested Loop
>>> import turtle
>>> turtle = turtle.Turtle()
>>> for n in range(36):
# repeat 6 times - move forward and turn
for i in range(6):
turtle.forward(100)
turtle.left(60)
turtle.right(10) # add a 10 degree turn (36 times) = 360 degrees
Background Color (add rainbow colors)
import turtle
colors = ['red', 'yellow', 'blue', 'orange', 'green', 'red']
shelly = turtle.Turtle()
turtle.bgcolor('black') #turn background black
***Naming shelly, turtle affect the code and had to rename shelly. Also indentation is very important here!! If it is off the shape can change or not draw as expected.
​
#make 36 hexagons, each 10 degrees apart
​
for n in range(36):
# make hexagon by repeating 6 times
for i in range (6):
shelly.color(colors[i]) #pick color at position i
shelly.forward(100)
shelly.left(60)
# add a turn before the next hexagon
shelly.right(10)
​
Add Circles around Pattern
# get ready to draw 36 circles
shelly.penup()
shelly.color('white')
# repeat 36 times to match the 36 hexagons
for i in range(36):
shelly.forward(220)
shelly.pendown()
shelly.circle(5)
shelly.penup()
shelly.backward(220)
shelly.right(10)
# hide turtle to finish the drawing
shelly.hideturtle()
Create a Row of Colored Squares
​
​
​
​
​
​
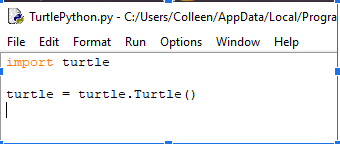
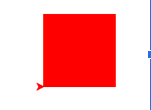

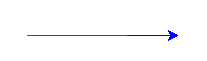

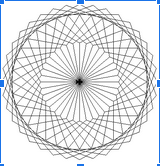

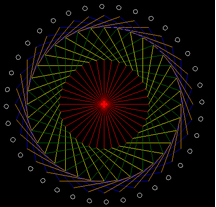